Randomness is required in a wide variety of different Android applications. For example, if you wanted your Android app to roll a die, flip a coin, or draw a card from a shuffled deck of cards, you will need some form of random number generation to achieve this.
Kotlin makes it really easy to generate random numbers to use in your Android app.
To generate a random integer within a range of two numbers, you can define an IntRange and then invoke the random() function on the IntRange which will return a random integer that falls within the range.
For example, if you wanted to generate a random integer between 1 and 10, you would use the following code.
var randomInteger = (1..10).random()
In the blog post below I will take you through some other ways to generate randomness in your Android app using Kotlin.
I will include code examples written in Kotlin that cover:
- How to generate a random integer (using a simulated dice roll as an example)
- How to generate a list of random integers (in just one line of code)
- How to generate a random double (in just one line of code)
- How to select a random item from a list (using a coin flip as an example)
- How to shuffle a list in random order (using a playing cards deck as an example)
All of the code shared in this tutorial is available on GitHub in the link below.
https://github.com/learntodroid/RandomNumbersKotlinAndroid
How to Generate a Random Integer in Kotlin
I will include some of the code shared in the introduction of this post for generating a random integer to use in an Android app that simulates rolling a 6-sided dice.
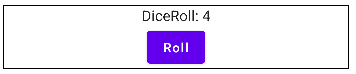
For the random number generation, I will create a Kotlin object called RandomSingleton that will be used as a singleton, meaning that only one instance of the object can be created and used throughout the app.
RandomSingleton will include a function called randomInteger that has two Int params which are the from and to values to be used for the range of the random number.
Then I will create an IntRange using those from and to numbers and call the random() function and return the random integer provided.
In the MainActivity, I will use a Jetpack Compose Composable function to display a Text element showing the rolled number and a Button to roll the dice
When the Button is clicked it will use the randomInteger function on the RandomSingleton passing the from and to values as 1 and 6 respectively which will return the number of a random dice roll.
The app will also display a Toast message with the random number after it has been rolled.
How to Generate a List of Random Numbers in Kotlin
In Kotlin, you can generate a list of random numbers between two numbers by using the random() function on an IntRange along with the map function.
See the code excerpt from the RandomSingleton object below containing the function listOfRandomNumbers which shows how to accomplish this in just one line of code.
To make use of the listOfRandomNumbers function in your MainActivity, see the code sample below that contains a Composable function called RandomList that takes in the size of the list and minimum and maximum values for the random numbers.
It displays the list of generated random numbers using the listOfRandomNumbers function from the RandomSingleton object inside a Text element with a Button displayed underneath. When the Button is clicked it will re-generate and show the random numbers.
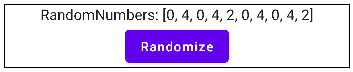
How to Generate a Random Double in Kotlin
In Kotlin, you can use the nextDouble function on the Random class to generate a random Double that falls within the range of two numbers.
The nextDouble function takes two parameters both of the type Double.
The random Double that is generated by the nextDouble function will be greater than or equal to the first parameter and will be less than the second parameter.
I have updated the RandomSingleton object to include a function for generating a random Double called randomDouble.
To use the randomDouble function in the RandomSingleton object in the Android app, see a code excerpt below from the MainActivity class.
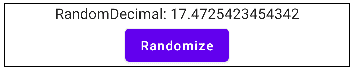
How to Select a Random Item from a List in Kotlin
In Kotlin, to retrieve a random item from a List all you need to do is call the random() function on a list and it will return a random item.
I have updated the RandomSingleton object to include a function called randomStringFromList that takes a List of Strings as a parameter and will return a random item from that list using the random() function.
This can be used in the MainActivity class with the following code excerpt.
In the MainActivity we are using the randomStringFromList function on the RandomSingleton object by passing a List of the two faces of a coin (heads and tails) then retrieving the random result, simulating a random coin flip.
The result of the coin flip is shown in a Text element and the user can flip the coin again by clicking the Button.

How to Shuffle a List in Random Order in Kotlin
In Kotlin, to randomize the order of a list, you can call the shuffle() function on a list.
I have updated the RandomSingleton object to include a function called shuffleListOfStrings that takes a MutableList of Strings as a parameter and will return the MutableList of Strings in a random order once it has been shuffled using the shuffle() function.
This can be used in the MainActivity class with the following code excerpt.
The code above uses the shuffleListOfStrings function on the RandomSingleton object to take in a MutableList of Strings which are made up of a list of playing cards that need to be shuffled.
The implementation for the Deck class is also provided below.
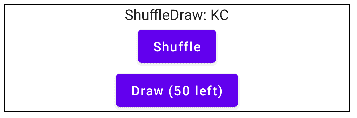