Android Studio requires you to have the Android SDK on your machine or you will not be able to create or build Android apps. Today when you do a fresh install of Android Studio it will come with the option to install the Android SDK.
An SDK, which stands for Software Development Kit, is a virtual toolbox used by programmers that contains software libraries you can reuse when coding an application rather than having to code everything from scratch saving effort.
The Android SDK offers a wide range of development tools for Android app developers which includes:
- Debugger
- Libraries
- Handset Emulator
- Documentation
- Sample Code
Tools in the Android SDK
Debugger
The debugger is available in Android Studio and can be used to you help locate and fix defects within your code.
In Android Studio you can set breakpoints in your code where you want to code execution to pause so that you can debug at that point in time. To create a breakpoint in Android Studio, find the line of code you want to set the breakpoint then left click to the right of the line number of the code and you should see a red circle appear.
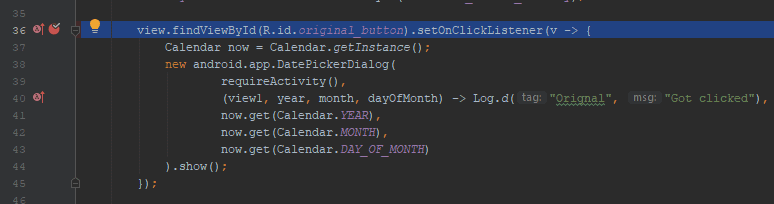
Once you have set you breakpoint, you can start debugging your application by selecting the debug icon, which looks like a green bug to the right of the run application button. This will launch your application in the emulator and proceed to pause the execution of your code at the line you have set your breakpoint.

You can use the debugger view at the bottom of Android Studio under the Debug tab to view the call stack of methods, as well as the current value of all the variables at that point in time. You can use the functions available in the Debug tab to step through subsequent lines in your code one step at a time, while observing the changes to the call stack and variables.
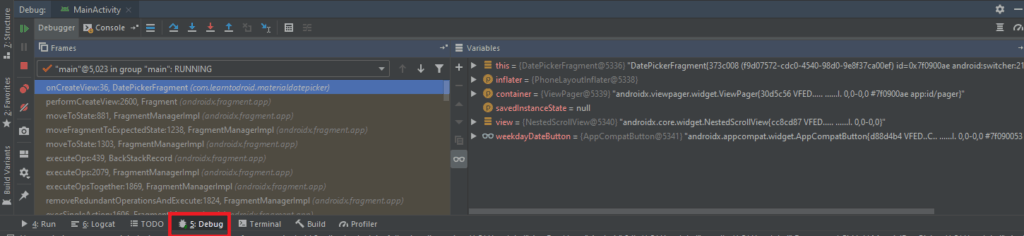
Libraries
The Android SDK includes many libraries that make your life easier as a software developer creating Android apps. The Android SDK libraries includes the Android support library and the AndroidX library. Use of the AndroidX library is recommended for all new projects.
Use the Android Developer website to find the list of packages under both the Android support library and AndroidX library which you can utilise by importing these packages using the import keyword at the top of your class referencing the package name.
For example, to import the Fragment class from the AndroidX library
import androidx.fragment.app.Fragment;
Emulator
The Android SDK comes with an emulator which is supported in the Android Studio IDE. The emulator allows you to interact with your Android apps on your local machine without needing to install your app on a physical handset, simplifying and speeding up the development process.
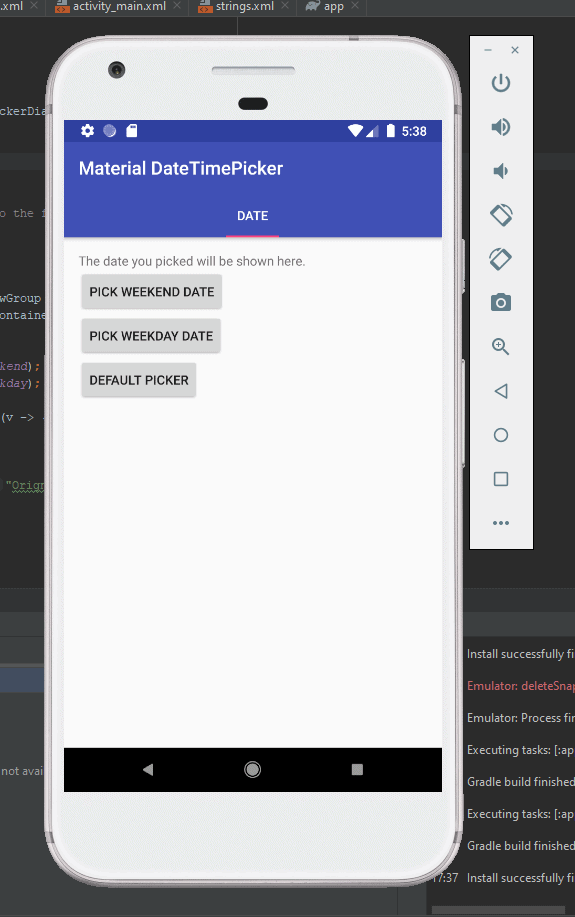
The emulator supports basic handset controls such as:
- Power button for powering the device on and off
- Volume up and down buttons
- Rotation of the screen to the left and right
- Taking a Screenshot and saving the screenshot to a location on your machine
- Zoom mode to zoom in and out
- Basic controls like back button, home button and overview button
The emulator also supports more advanced handset controls such as:
- Modifying location details by passing GPS coordinates
- Setting cellular network details such as network type or signal strength
- Changing the battery attributes such as charging status and charge level
- Making phone calls to the device or sending SMS messages
- Microphone settings to use voice related functions on your virtual device through a microphone connected to your machine
- Passing pre-created fingerprints to the device
- Manipulation of device sensors such as accelerometer, gyroscope, magnetometer and ambient temperature
The Android SDK comes with an Android Virtual Device (AVD) Manager accessible through Android Studio. The AVD Manager allows you to create virtual devices that are run by the handset emulator.
You can create a wide range of virtual devices across different categories including Android TVs, Phones, Tablets and Wear OS to use on the emulator.
Documentation
Using the Android SDK Manager in Android Studio you can download the Android SDK documentation to your local machine by following the instructions below.
- Select the “Tools” menu
- Select the “SDK Manager” menu item
- Navigate to the “SDK Tools” tab
- Check the “Documentation for Android SDK” check
- Select “OK” and wait for the download and installation to complete
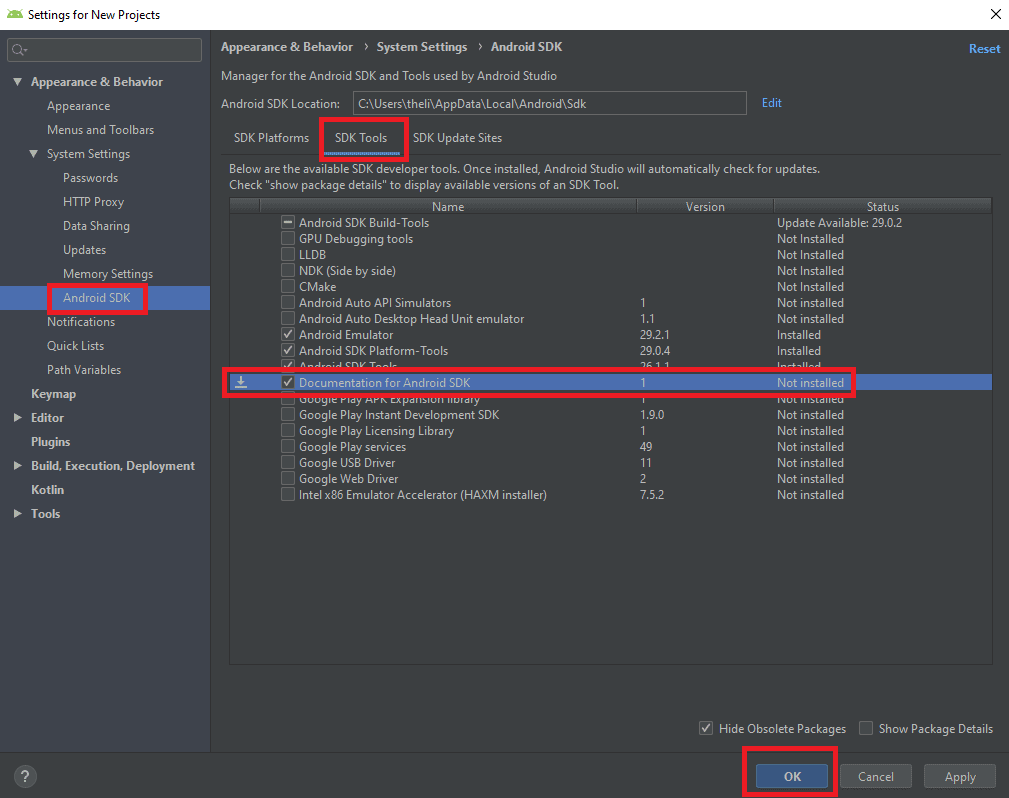
To access the documentation when in Android Studio, highlight the method name or type and press the “Shift” and “F1” keys at the same time which will open the documentation based on the selection in the web browser.
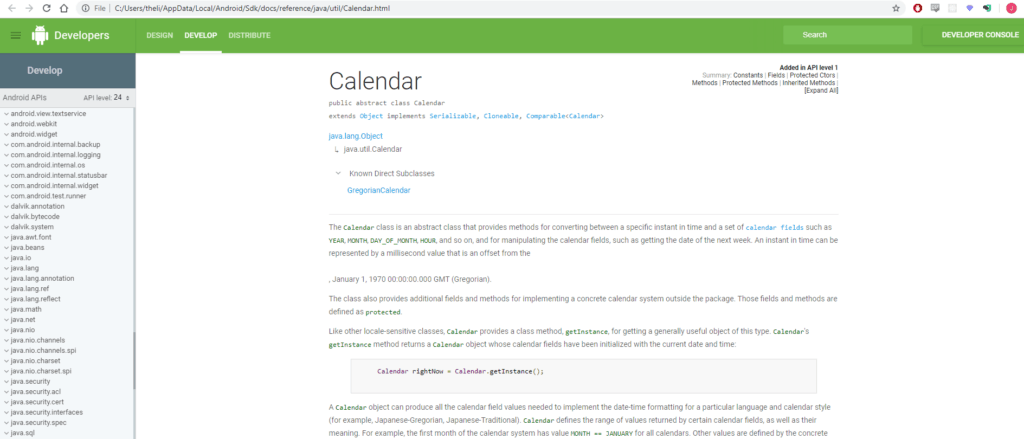
To view the same documentation in Android Studio, highlight the relevant text then press the “Ctrl” and “Q” keys for Windows or “F1” for Mac.
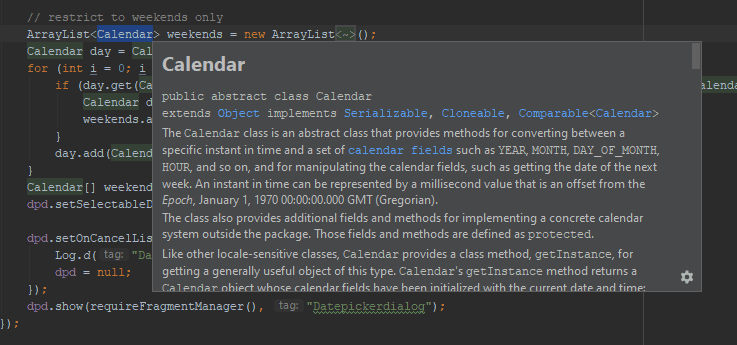
To find the full set of documentation to view in your browser, navigate to your Android SDK path (see “How can I find the Android SDK path in Android Studio” section bellow) and open up the index.html file into the web browser which is located in the “docs” folder within Android SDK directory
Sample Code
The Android SDK offers a large range of sample code you can import into your project. You can browse the samples that have been created in Android Studio then import them into a new project.
Some of the examples of sample code available today include:
- Android Things samples
- Animation samples
- Instant App samples
- Graphics samples
- Background tasks samples
- Camera samples
To import sample code into a new project in Android Studio
- Select the “File” menu, Navigate to the “New” Menu, Select the “Import Sample” Menu item
- Browse the samples and select one you like
- Select the “Next” button
- Fill out the Application Name and Project Location
- Select the “Finish” button
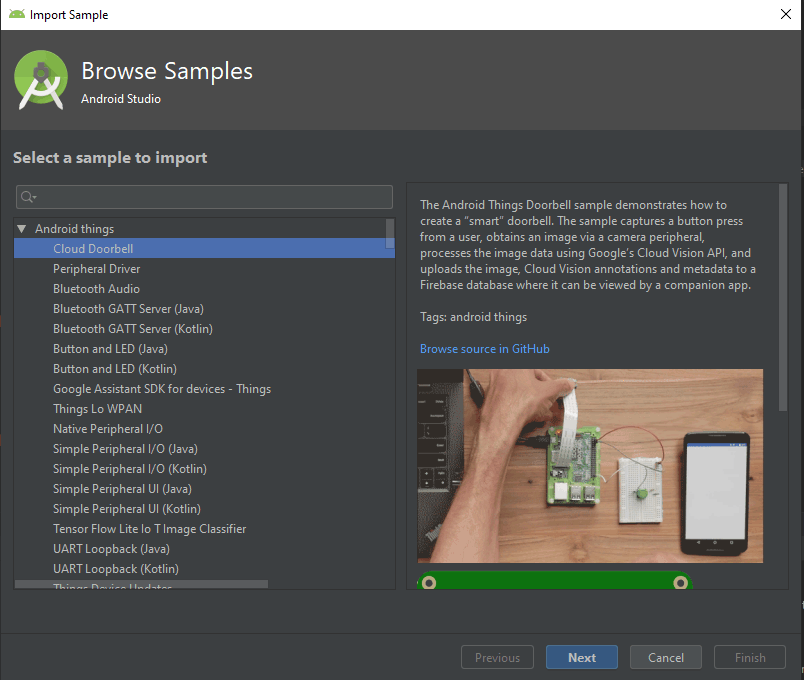
How can I find the Android SDK path in Android Studio?
If you want to find out the location of the Android SDK that is being used by Android Studio on your computer, follow the steps below the find the Android SDK path.
To find the Android SDK path in Android Studio:
- Open Android Studio
- Select the “File” menu
- Select the “Settings” menu item
- Use the navigator on the left hand side and select “Android SDK” located under “Appearance & Behavior”, “System Settings”, “Android SDK”
- Android SDK Location field will show the path on your computer to the Android SDK
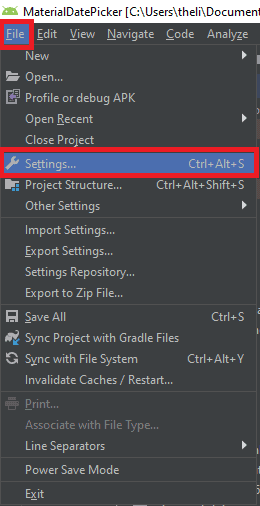
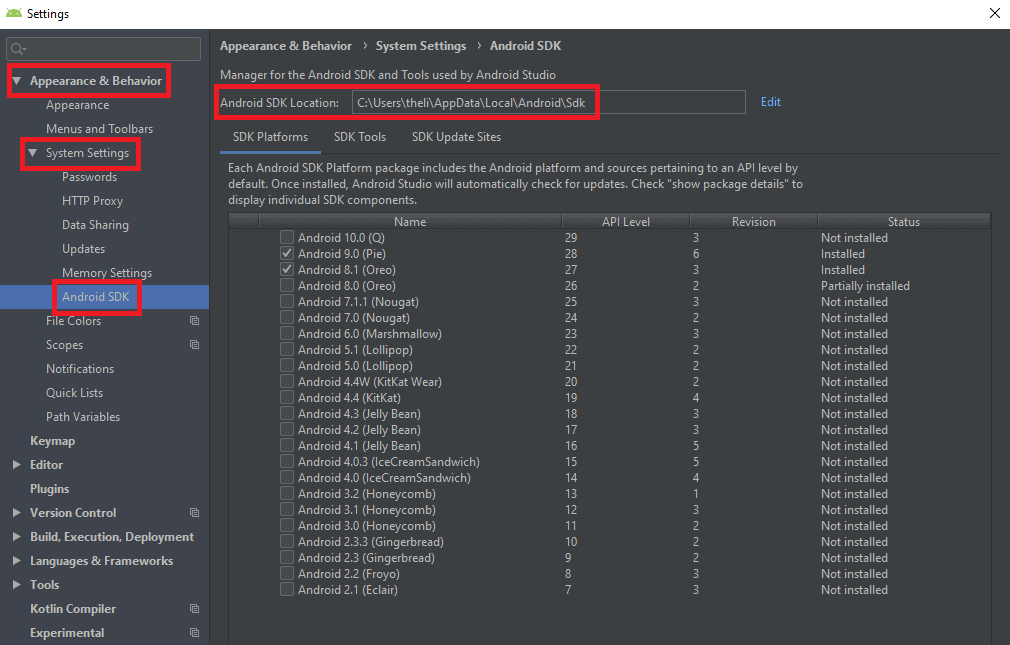
How to change the Android SDK path in Android Studio
- Open Android Studio
- Select the “File” menu
- Select the “Settings” menu item
- Use the navigator on the left hand side and select “Android SDK” located under “Appearance & Behavior”, “System Settings”, “Android SDK”
- Select the Edit link to the right of the Android SDK Location field and browse your local machine to select a different path to the Android SDK
How does licensing work with the Android SDK?
The terms of the license agreement for the Android SDK are available on the Android developers website. You will need to accept the license agreement in order to use the Android SDK.
Reviewing and accepting the license agreement for the Android SDK will be triggered during the installation process.
You can also accept the license agreement for the Android SDK in the command line using the sdkmanager tool with the following command.
yes | sdkmanager --licenses
How can I upgrade the Android SDK in Android Studio?
Upgrades to the Android SDK can be managed in Android Studio using the SDK Manager accessible via the “File” menu under “Tools”, “SDK Manager”.
In the SDK Platforms tab you can trigger upgrade to the newest revision of existing SDK Platforms you have downloaded or an entirely new SDK Platform.
New versions of the Android SDK are releasing on roughly a yearly basis.
- Android 10.0 (Q) was released in September 2019
- Android 9 (Pie) was released in August 2018
- Android 8.0 (Oreo) was released in August 2017
What are the differences between Android SDK build tools, platform tools and tools?
Name | Description | Category |
Emulator | Used for running your Android app on a virtual device | tool |
ProGuard | Code optimization | tool |
mksdcard | Create a FAT32 disk image for use in the emulator | tool |
avdmanager | Create and manage Android Virtual Devices | tool |
sdkmanager | View, install, update, and uninstall packages for the Android SDK | tool |
jobb | Tool for building OBB files that are used for supplying assets such as graphics, video and audio for Android apps and kept separate from the APK | tool |
APK Signer | Sign your APK | build tool |
D8 | Compiles Java bytecode into dex files | build tool |
DX | Compiles Java bytecode into dex files | build tool |
adb | Android Debug Bridge used to communicate with devices | platform tools |
dmtracedump | Tool that generates graphical call-stack diagrams from trace log files | platform tools |
etc1tool | encode PNG images to the ETC1 compression standard and decode ETC1 compressed images back to PNG | platform tools |