As a part of the series for creating apps from scratch, I have put together a tutorial on how to build a BMI calculator app for Android.
In this tutorial, we will build a BMI calculator app for Android that:
- Captures weight and height with EditText widgets
- Contains a class that uses the Singleton Pattern and contains the calculation logic for BMI
- Uses a ToggleButton to support calculation of BMI with both the metric and imperial measurement systems
- Displays the calculated BMI and categorization of the BMI inside a CardView widget
All of the code samples shared in this post are available in GitHub.
Creating a BMI Calculator Android App Tutorial
In this tutorial, we will be creating an Android app that calculates Body Mass Index (BMI) based height and weight entered by the user.
We will support both the metric unit system and the imperial unit system for calculating the BMI which allows the user to enter weight in either kilograms or pounds and the height in centimeters or feet and inches.
See the sample screenshots below for the Android app we will build.
Creating a New Android Project
We will start this tutorial for building a BMI calculator by creating a brand new project in Android Studio.
Open Android Studio and follow the wizard for creating a new Android project. Select the “Blank Activity” project template and provide a project name, set the package name then select “Finish” to create the Android project.
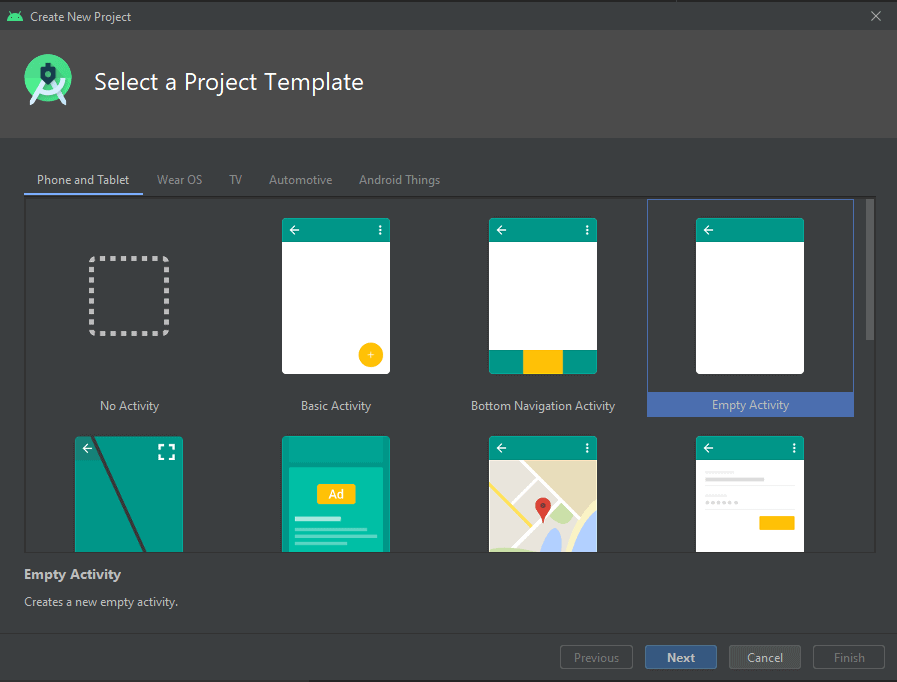
Removing the Action Bar
Once the Android project has been created, open the styles.xml resource file in the /app/src/main/res/values directory and update the parent attribute on the style element to value “Theme.AppCompat.Light.NoActionBar” to remove the Action Bar from the Activity.
See a sample of the styles.xml resource file that has removed the Action Bar below.
Setting up the Activity Layout Resource
We will be using a single Activity for the BMI calculator app.
In the layout resource file for this Activity activity_main.xml, we will add the following user interface elements inside a LinearLayout with a vertical orientation.
First, we will add a TextView inside the LinearLayout, that will display the title of the app as “BMI Calculator”. We will center the TextView horizontally by setting the “android:gravity” attribute to “center_horizontally”.
Then, we will add a ToggleButton that we will use for toggling between the metric system and the imperial system of measurements for weight and height. We will default it to the metric system by setting the “android:checked” attribute value to “true” and setting the “android:textOn” attribute value to “Metric Units” and “android:textOff” to “Imperial Units”.
Next, we will add LinearLayout with a horizontal orientation and we will place two CardView widgets that will sit next to each other. These CardView widgets will be used for populating the height and weight information.
Both will the following attribute values populated to give the the CardView widgets rounded corners with a margin in between:
- “android:layout_margin” set to “6dp”
- “app:cardElevation” set to “6dp”
- “app:cardCornerRadius” set to “6dp”
The CardView on the left side will contain the height information. There will be TextView with the text of “Height” centered inside the CardView.
Underneath the TextView there will be an EditText for capturing the height in centimeters which only accepts decimal numbers via the attribute value of “android:inputType” set to “numberDecimal”.
There will also be a LinearLayout with a horizontal orientation containing two EditText widgets for capturing the height in feet and inches.
Later inside the Main Activity class, we will control the display of these EditText widgets based on whether the user has chosen to use the metric measurement system or the imperial system using the ToggleButton.
The CardView on the right side will contain the weight information. There will be TextView with the text of “Weight” centered inside the CardView.
Underneath that TextView there will be two EditText widgets that also only accept decimal numbers that will be used for capturing the weight in kilograms or in pounds.
Next, we will add a Button with the label of “Calculate” which when selected will calculate the BMI and display the results underneath.
For that last part of the Activity layout we will add some UI elements for displaying the calculated BMI results.
We will add a CardView widget that will take up the full width of the screen.
Inside this CardView, we will show a TextView with the text of “BMI” centered inside the CardView.
Underneath this TextView, we will add another TextView that will be used for displaying the calculated BMI value.
Then finally, we will have one more TextView that will display the category of the BMI calculated. These categories include “Underweight”, “Healthy Weight”, “Overweight” and “Obese”.
Implement a Class for Calculating BMI
In this section of the tutorial, we will create a new class that will contain methods used for calculating BMI in both imperial and metric units.
We will be creating this new class to separate the logic from calculating the BMI outside of the MainActivity logic that will be used for setting up the user interface and responding to events that occur in the UI.
We will give this new class the name “BMICalcUtil” and we will follow a singleton pattern where we will initialize a single instance of the BMICalcUtil and assign it to a class variable. We will then use a getter method called getInstance to retrieve this BMICalcUtil instance from within the MainActivity class.
We will add three more methods to the BMICalcUtil class.
Two of these methods will be used for calculating the BMI in the metric and the imperial unit system using the formulas below.
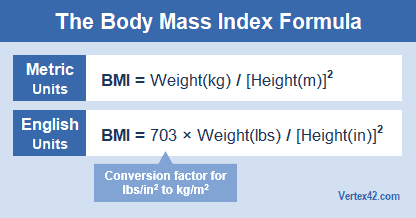
The third method will be used for classifying the BMI value as either “Underweight”, “Healthy Weight”, “Overweight” and “Obese” based on the table below.
BMI | Category |
---|---|
Less than 18.5 | Underweight |
Greater than or equal to 18.5 and less than 25 | Healthy Weight |
Greater than or equal to 25 and less than 30 | Overweight |
Greater than or equal to 30 | Obese |
See a code sample of the BMICalcUtil class below.
Setting up the Main Activity Class to Calculate and Display BMI Results
In this last section of the tutorial, we will update the MainActivity class to retrieve height and weight measurements entered by the user and to interact with the BMICalcUtil class to calculate then display the BMI results.
In the onCreate method, we will use the findViewById method to initialize class variables for the various UI elements we have added to the Activity layout resource.
These UI elements include:
- EditText widgets for capturing the weight and height measurements in both measurement systems
- A ToggleButton for switching between the metric and imperial unit system
- A Button for calculating the BMI results
- A CardView widget that will contain two TextView widgets for displaying the BMI result and category
Then, in the onCreate method we will enable the metric system by default by setting the inMetricUnits boolean class variable to true.
After that, we will invoke the updateInputsVisibility method we will add to the MainActivity class that is responsible for ensuring the correct EditText widgets are displayed on screen based on the measurement system that is being used.
Also in the onCreate method, we will hide the CardView widget used for displaying the BMI results by using the setVisibility method and passing the View.GONE value as a paramater.
Then, we will set OnClickListeners to the Button used for calculating the BMI results and the ToggleButton for switching measurement systems.
Inside the OnClickListener for the ToggleButton in the onClick method we will toggle the value for the inMetricUnits boolean variable then call the updateInputsVisibility method to display the relevant EditText widgets on screen.
In the onClick method for the OnClickListener on the Button used for calculating the BMI results we will first check which measurement system is being used.
Then we will check that those EditText widgets that relate to the selected measurement system are not blank. If any of the applicable EditText widgets are blank a Toast message will be shown to the user advising them to populate the weight and height information.
After that, the values from the EditText widgets will be passed the BMICalcUtil by invoking either the calculateBMIMetric or calculateBMIImperial methods.
The BMI result will then be provided as a parameter to the displayBMI method which will show the BMI results CardView and update the TextView widgets inside the CardView with the BMI and the category.
See a code sample of the MainActivity class below.