The material design library for Android offers two components MaterialButtonToggleGroup and MaterialButton that can be used to create a group of buttons can toggled on or off while having only a single button being selected at any time.
See an example of the MaterialButtonToggleGroup and MaterialButton widgets used below to select the period of data for stock prices to display in a chart in an Android app.

In this post I will show you, with code samples written in Java (also available in GitHub), how to use the MaterialButtonToggleGroup and MaterialButton widgets from the material design library for Android to create a group of buttons that can be toggled on and off.
How to use the MaterialButtonToggleGroup and MaterialButton Widgets in Android
In this section of the tutorial on using the MaterialButtonToggleGroup and MaterialButton widgets I will show you code samples on how to create a set of buttons that can be toggled.
Step 1: Retrieve the Android Material Design Dependencies using Gradle
First we will need to add the implementation dependency for the Android material design library to our Android project in order to use the MaterialButtonToggleGroup and MaterialButton widgets.
In your build.gradle file inside in the app directory in your Android project, add the following dependency to get access to the MaterialButtonToggleGroup and MaterialButton widgets.
Step 2: Setup the theme in the styles.xml file and the Android App Manifest
Next we will need to set up the them in the located in the styles.xml file to use with your Android app.
For the style element used for as the theme for your Android app in your resource style.xml file, make sure you have a parent attribute set to “Theme.MaterialComponents” as per the code sample below.
In the Android app’s manifest file, the application element will need to reference the theme we have created in the android:theme attribute as per the sample manifest file below.
Step 3: Add the MaterialButtonToggleGroup and MaterialButton Widgets to the Fragment or Activity Layout Resource File
Next we will update the layout resource we will use for our fragment or activity and add the MaterialButtonToggleGroup and the MaterialButton widgets.
In the layout resource, we will first add a MaterialButtonToggleGroup widget. In the MaterialButtonToggleGroup widget we will add the XML attribute “app:singleSelection” with the value “true” to enforce only a maximum of one button in the MaterialButtonToggleGroup to be selected at any at time.
Inside the MaterialButtonToggleGroup widget we will add three MaterialButton widgets in the layout resource. On each of the MaterialButton widget we will add the following attributes.
- A unique id as the value of the “android:id” attribute
- An “android:text” attribute with the value set to the label of the button
- A “style” attribute with the value “?attr/materialButtonOutlinedStyle”
See a sample layout resource below for an activity containing the MaterialButtonToggleGroup widget with three MaterialButton widgets inside.
Now if you run your Android app you should see something like the following screen capture recording.
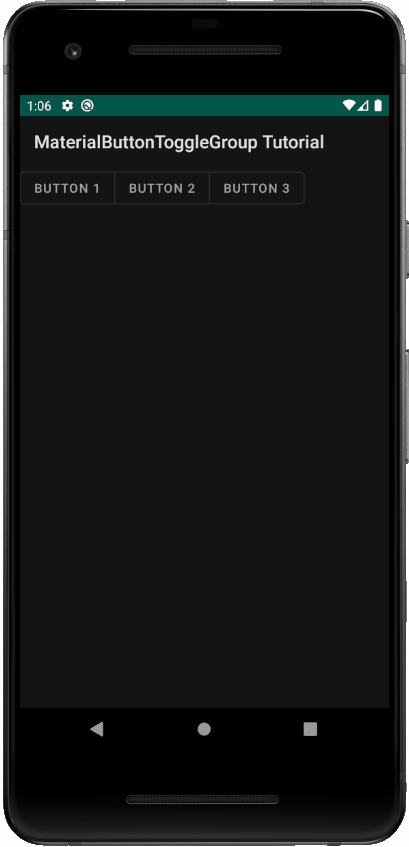
How to Set the Selected MaterialButton in MaterialButtonToggleGroup
There are two ways you can set the button that is checked in the MaterialButtonToggleGroup.
- Option 1: using the checkedButton XML attribute on the MaterialButtonToggleGroup
- Option 2: using the check(int) method on the MaterialButtonToggleGroup
Checked Button XML Attribute on MaterialButtonToggleGroup
The MaterialButtonToggleGroup widget has an XML attribute named “app:checkedButton” that can be used to load a button in the group as checked. To set a particular button inside the MaterialButtonToggleGroup to load as checked by default, pass the id of the button to be checked as the value to the “app:checkedButton” attribute.
See the code sample below showing the second MaterialButton loading as checked in the MaterialButtonToggleGroup.
Check Method on MaterialButtonToggleGroup
The MaterialButtonToggleGroup widget has a method check(int) which can be used to check a MaterialButton programmatically by passing the id of the MaterialButton as a parameter.
See the code sample below using the check(int) method on the MaterialButtonToggleGroup to check the third MaterialButton in the MaterialButtonToggleGroup.
How to Clear the Selected MaterialButton from MaterialButtonToggleGroup
There are two ways to clear a selected MaterialButton from a MaterialButtonToggleGroup programmatically in Android.
The first way involves using the uncheck(int) method on the MaterialButtonToggleGroup passing the id of the MaterialButton to be unchecked as a parameter.
The second approach is to use the clearChecked() method on the MaterialButtonToggleGroup.
How to Get the Selected MaterialButton from a MaterialButtonToggleGroup
If the MaterialButtonToggleGroup is in single selection mode (which can be set using the “app:singleSelection” XML attribute or by invoking the setSingleSelection(boolean) method on the MaterialButtonToggleGroup) the id of the selected MaterialButton can be retrieved using the getCheckedButtonId() method on the MaterialButtonToggleGroup.
If there is no selected MaterialButton the getCheckedButtonId() method will return the NO_ID value.
See the code sample below showing how to get the selected MaterialButton from a single selection MaterialButtonToggleGroup using the getCheckedButtonId() then creating and showing a toast of the selected MaterialButton text.
If the MaterialButtonToggleGroup has multi selection enabled, the getCheckedButtonIds() method on the MaterialButtonToggleGroup can be used to retrieved a list of ids of the MaterialButtons that are selected in the MaterialButtonToggleGroup.
See the code sample below showing how to get the ids of each of the selected MaterialButtons in the MaterialButtonToggleGroup and showing a toast containing a concatenated string of each of the selected MaterialButton text labels.
How to Mandate that a MaterialButton is Always Selected in a MaterialButtonToggleGroup
There are two ways to ensure a MaterialButton is always selected in a MaterialButtonToggleGroup.
The first involves setting the “app:selectionRequired” XML attribute on the MaterialButtonToggleGroup to “true”.
See an example of a layout resource using the “app:selectionRequired” XML attribute on the MaterialButtonToggleGroup to require at least one MaterialButton to be selected.
Alternatively you can use the setSelectionRequired(boolean) method on the MaterialButtonToggleGroup passing true as the parameter.
How to Fix Error Inflating Class when using MaterialButton and MaterialButtonToggleGroup
You may experience an error when inflating a view containing a MaterialButton and MaterialButtonToggleGroup.
See the logs below of an example of the error inflating a view containing a MaterialButton and MaterialButtonToggleGroup widgets.
To fix this error make sure the style used in your Android application has the parent attribute value of “Theme.MaterialComponents” in the styles.xml file.
See a sample styles.xml resource below.
Also make sure this style is referenced correctly in your Android app’s manifest file in the android:theme attribute of the application element as per the sample manifest file below.