At the moment I am working on app to help keep me motivated on my goals by using a count down timer to track the time I spend on each of my goals everyday. I have put together this tutorial with what I have learnt on creating a count down timer in an Android app using Java.
To create an Android app with a count down timer follow the steps below:
- Set up a user interface to capture the time in hours, minutes and seconds you wish to count down from
- Set up a user interface to show the time remaining in hours, minutes and seconds before the count down completes with a button to start the timer
- Use a CountDownTimer to set up the timer that starts when the start button is pressed, providing the time the count will last for and the interval you will update the screen with changes to the count. Override the onTick(long) method to handle screen updates at a set internal and override the onFinish() method to handle the count down expiry
- (Optional) Add additional functionality count down timer to pause, resume and reset the timer
What functionality does the CountDownTimer offer?
- The CountDownTimer was added in Android API level
- It is available in the android.os package and can be imported using “import android.os.CountDownTimer;”
- The constructor for CountDownTimer takes two arguments
- The first argument in the constructor, long millisInFuture, is the number of milliseconds in the future after the count down timer is started (using the start() method) that the timer will expire and trigger the onFinish() callback
- The second argument in the constructor, long countDownInternal, is the interval (in milliseconds) when the onTick(long) callback will be triggered
- The CountDownTimer has a start() method to begin the timer and a cancel() method to end the timer
- When creating a CountDownTimer to use, the onTick(long) and onFinish() methods will be overriden
- Overriding the onTick(long) method will allow you to trigger specific functionality, such as updating the remaining time left in the count down, every time the interval elapses
- Overriding the onFinish() method will allow you to trigger specific functionality once the count down has completed, for example, showing a count down completion message
Creating an App using CountDownTimer
We will be making a simple count down timer Android app written in Java in this tutorial. The code for this tutorial is available publicly in the Count Down Timer repo in GitHub.
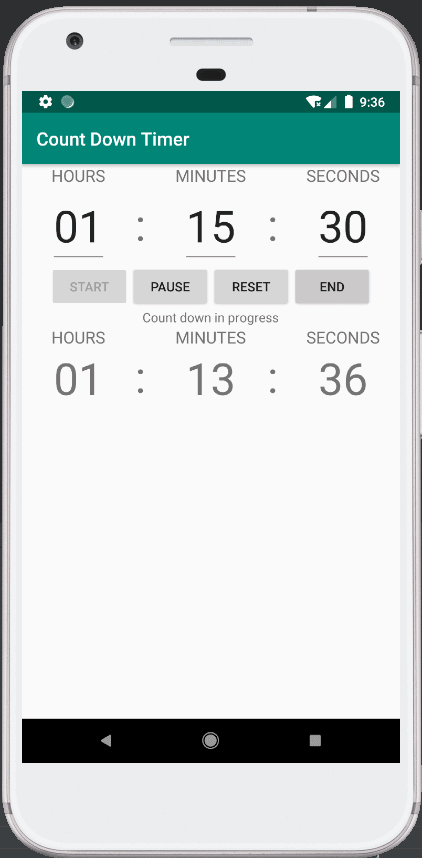
Step 1: Capturing the Count Down Time
In this Count Down Timer app, we will support the ability to count down from a particular number of hours, minutes and seconds in the future.
Add three EditTexts in the user interface to capture the hours, the minutes and the seconds we want to count down from. Put a TextView above the hours, minutes and seconds to describe the units being entered. Between the EditText for hours and minutes and the EditText between minutes and seconds place a TextView with the colon “:” symbol.
Below is an excerpt from the layout file for the MainActivity activity_main.xml which shows the layout for capturing the time we will be counting down from.
Step 2: Displaying the Count Down
Below the location where we will be capturing the time we are counting down from will be a set of buttons that will trigger the starting, pausing, resuming, resetting and ending of the timer.
Underneath those buttons we will show the current state of the count down in a TextView. Beneath that, we will show count down time remaining broken down by hours remaining, minutes remaining and seconds remaining until the count down will elapse.
Below is an excerpt from the layout file for the MainActivity activity_main.xml which shows the layout for displaying the current state of the count down and the time remaining in the count down.
Step 3: Creating and Starting the Count Down Timer, Handling Expiry and Cancellation
We will be adding two buttons to the user interface that will be used to perform the following functions on the count down timer
- The Start button will start the count down timer from the time entered by the user
- The End button that will cancel the count down timer
Below is an excerpt from the layout file for the MainActivity activity_main.xml which shows the layout for the buttons that will be used to drive the count down timer.
Below is an excerpt from the MainActivity class which includes code for setting up two buttons, a button to start the timer and a button the end the timer.
The start timer button has an on click listener, which when clicked performs the following:
- Calculate the time in the future it will count down from by converting the hours, minutes and seconds captured on the count down into milliseconds then adding them together
- Disable the start timer button and enable the end timer button
- Instantiate a CountDownTimer providing the total milliseconds it will count down to and an interval of 1000 milliseconds (i.e. 1 second) to update the user interface
- The onTick(long) method has been overridden and will be called at the interval of every 1 second, until the count down finishes or is cancelled, to update the remaining time in the count down in the user interface
- The onFinish() method has been overriden and will be called once the count down elapses to show a count down completion message in the user interface and enable the start timer button and disable the end timer button
- The CountDownTimer will start counting down immediately after it has been instantiating through the use of the start() method
The end timer button has an on click listener, which when clicked performs the following:
- Cancel the CountDownTimer using the cancel() method
- It will update the user interface to show a timer cancellation message and will enable the start timer button and disable the end timer button
Step 4 (Optional): Supporting Pause, Resume and Reset of the Count Down Timer
This step is optional, we will be adding some functionality to the app to let the user pause the count down that has been started and resume from where it was paused. In addition to this we will include the option to reset the count down timer to the state it was when the count down timer was started.
Below is an excerpt from the MainActivity class which includes code for handling the button press for the reset timer button and the pause / resume timer button.
The reset timer button has an on click listener, which when clicked performs the following:
- Disables the start timer button and enables the reset and pause buttons
- Sets the pause button to the pause toggle (rather than resume) as the count down timer will be running
- It will cancel the existing count down timer running and will create a new timer using the count down provided when the timer was last started
The pause / resume timer button has a listener, which when clicked performs the following:
- Toggle between pause mode or resume mode
- If the user selected pause, it will cancel the count down timer and show a message in the user interface that the count down timer has been paused
- If the user selected resume, it will create and start a new count down timer using the count down time remaining when the timer was paused
Related Questions
How can I get the Count Down Timer to work in the Background?
To achieve this you will want to use a background service, which is beyond the scope of this post, but will allow you to continue to count down the timer while it is not currently on screen.
You may also want to consider showing the count down status and remaining time of the count down timer in a notification while the app is not in focus.
How can I trigger vibration when the Count Down Timer elapses?
You can get the device to vibrate when the count down timer expires by use of the Vibrator and the VibrationEffect classes by calling the Vibrator’s vibrate(VibrationEffect) method within the overriden onFinish() method on your CountDownTimer.
You can use the createWaveForm(long[], int) method on the VibrationEffect to generate a repeating vibration.
You will need to add the Manifest.permission.VIBRATE permission in your Manifest file in order to achieve this.
How can I add a visual progress bar to the Count Down Timer?
The first thing you will need to do is to calculate the % of progress completed. This will need to be done in the onTick(long) method using the following calculation.
double progress = 1.0 - (millisUntilFinished / countDownTimeInMilliseconds)
A good way to show the remaining time in a progress bar form would be using a circular progress bar. There isn’t a circular progress bar available out of the box with Android. You have the options to create your own custom version of a circular progress bar, otherwise consider a using one of the reusable circular progress bars for Android from GitHub.