Adding video content to your Android app can create a great interactive experience for your users. YouTube offers a massive library of videos and a reliable video streaming platform that you can leverage to embed video into your Android app.
YouTube offers an official API to allow developers to play YouTube videos in their app, however, it has not been updated in a long time and it requires the user to have the YouTube app installed on their device.
A great alternative free to use, open-source API that allows you to embed YouTube videos to be played within an Android app is android-youtube-player created by Pierfrancesco Soffritti.
android-youtube-player uses a WebView with an IFrame to display and expose the controls to the YouTube player. In addition to being able to play videos from YouTube, android-youtube-player also you to customize the user interface of the video player as well as offering the ability to cast YouTube videos from the player to a Chromecast device.
In this post I will show you how to set up and the android-youtube-player library in your Android project and play YouTube videos embedded into your app.
The code samples showed in this tutorial are available on GitHub in the following repository.
https://github.com/learntodroid/AndroidEmbedYouTubeVideoTutorial
A video tutorial for this post is available in the embedded YouTube video below.
Android-Youtube-Player Tutorial
Retrieve the Android-Youtube-Player Dependency using Gradle
To obtain the latest Gradle dependency for the android-youtube-player API check out the GitHub repository for the project at the link below.
https://github.com/PierfrancescoSoffritti/android-youtube-player
In the Download section of the README.md you will find the Gradle dependency.
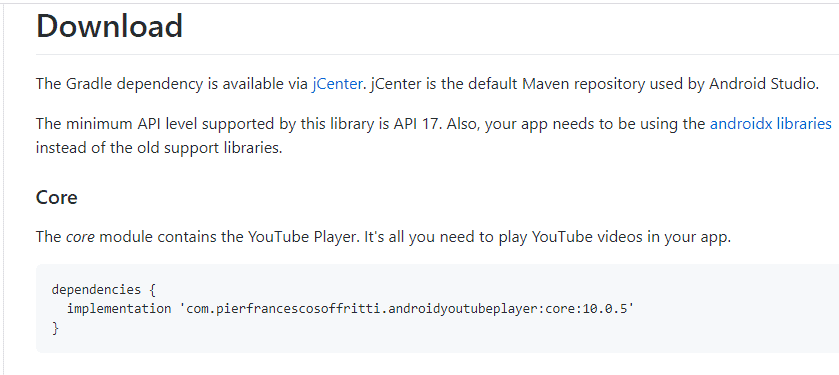
We will add this dependency to the app level build.gradle file in your Android project.
While we update this dependency in the gradle file, this API requires a minimum API level of 17, update the minSdkVersion in the default config section of the app level build.gradle file to API level version 17 or above.
See a sample app level build.gradle file below.
Add the INTERNET Permission in the Manifest
As we will be loading YouTube videos from the Internet we will require the INTERNET permission to be added in the app’s manifest file.
See a sample Android app manifest file containing the INTERNET permission.
Play a YouTube Video using the Layout Resource
In this section of the tutorial, we will use the layout resource file for the Activity to add the YouTubePlayerView widget and get it to play automatically using XML attributes on the YouTubePlayerView.
For the purposes of this tutorial, we will use the YouTube video below from the Android Developers YouTube channel introducing the Android operating system with a demo.
Next, we will update the Activity layout resource to add the YouTubePlayerView widget.
We will set the app:videoId XML attribute to the YouTube video ID found in the URL of the YouTube video as per the image below.
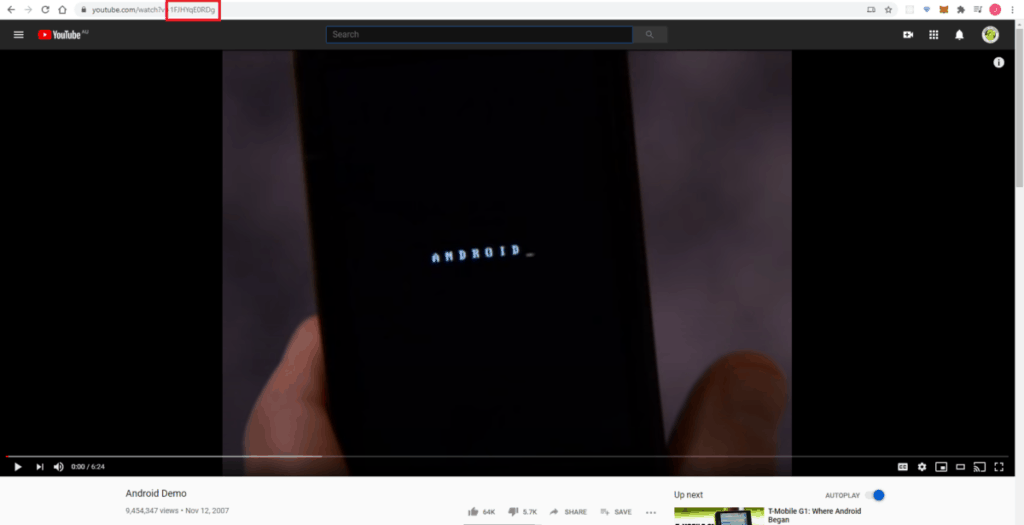
We will enable autoplay on the video when the Activity is displayed by setting the app:autoPlay XML attribute to true. We will also enable the full-screen button on the YouTubePlayer widget by setting the app:enableFullScreenButton XML attribute to true.
See a sample Activity layout resource file with these details populated below.
For the full list of XML attributes available for the YouTubePlayerView widgets follow the links below to the documentation available in the GitHub repository.
- videoId
- autoPlay
- enableAutomaticInitialization
- handleNetworkEvents
- useWebUi
- enableLiveVideoUi
- showYouTubeButton
- showFullScreenButton
- showVideoCurrentTime
- showVideoDuration
- showSeekBar
Next, we will need to update the Activity class the add the YouTubePlayerView widget as a lifecycle observer to the Activity. Adding the lifecycle observer will automatically pause the YouTube video when the Activity stops.
See the code sample below for the Activity class.
Play a YouTube Video Programmatically
The android-youtube-player library also allows you the play a YouTube video programmatically in your Android app.
To do this in the Activity or Fragment you will need to add an AbstractYouTubePlayerListener to the YouTubePlayerView widget.
Inside the onReady(…) method of the AbstractYouTubePlayerListener you can autoplay the YouTube video by calling the loadVideo(…) method on the YouTubePlayerView widget passing the YouTube video ID as the first parameter and the start time as the second parameter.
If you want the YouTube to be cued up instead of autoplaying, call the cueVideo(…) method on the YouTubePlayerView widget passing the YouTube video ID as the first parameter and the start time as the second parameter.
Further Reading
https://medium.com/@soffritti.pierfrancesco/customize-android-youtube-players-ui-9f32da9e8505
https://github.com/PierfrancescoSoffritti/android-youtube-player