If you ever need to show a scrollable list of items in your app you might consider using a RecyclerView or ListView to achieve this. In this article I will summarise the major differences between RecyclerView and ListView in Android.
The key differences between the RecyclerView and ListView in Android are documented in the table below.
Criteria | RecyclerView | ListView |
---|---|---|
Requires additional dependency | Yes, RecyclerView requires an additional dependency | No, available natively |
ViewHolder Pattern | Mandatory ViewHolder pattern providing better performance | ViewHolder pattern can be supported with customisation |
Layout Management | Advanced Layout Management capabilities for vertical and horizontal lists, grids and staggered grids | Vertical list only |
Adapters | Supports notifications if the entire data set has changed, item gets added, item gets removed or item gets changed | Supports notifications if the entire data set has changed |
Item Decorations | Dividers between items not shown by default. Use ItemDecorations to add margin and draw on or under an Item View. | Dividers between items shown by default. Item decoration requires customisation for ListView. |
Item Animation | ItemAnimator makes it easy to add animations | Very complex to implement |
Official Documentation | RecyclerView documentation | ListView documentation |
In the sections below I will describe in the greater detail the functionality available in RecyclerView and ListView APIs. In the Conclusion section I will summarise the key differences between RecyclerView and ListView and in describe in what circumstances each should be used.
What is a RecyclerView?
A RecyclerView is used to display a large list of items with their own specific Views within the user interface in a performance optimised manner.
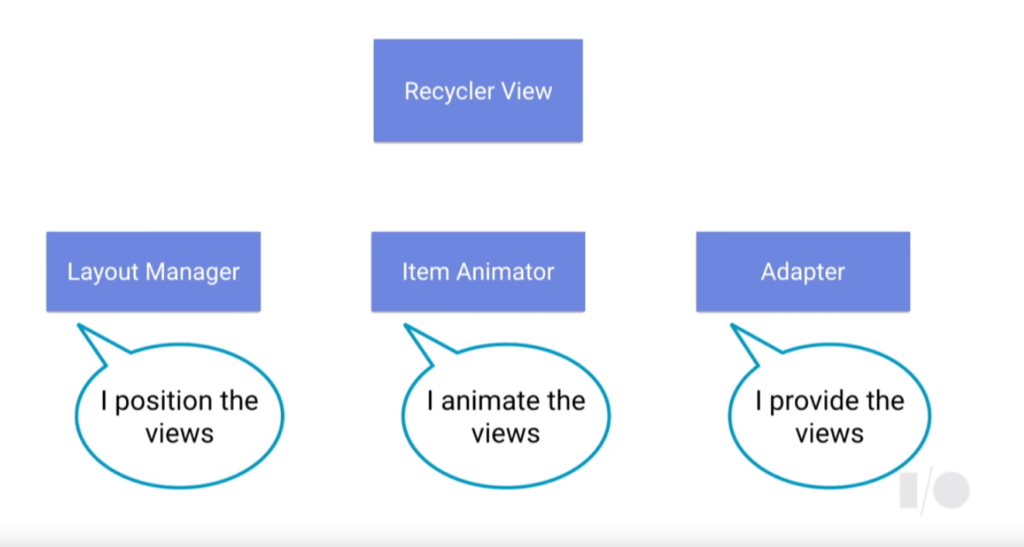
Credits: RecyclerView ins and outs – Google I/O 2016
LayoutManager
The RecyclerView uses a LayoutManager to determine how the Views will be placed within the RecyclerView. The different LayoutManagers supported by RecyclerView include:
- LinearLayoutManager, a vertical or horizontal list of items
- GridLayoutManager, a grid of items that are all the same size
- StaggeredGridLayoutManager, a grid of items that supports different sizes
ViewHolder
In a RecyclerView, your items will have a specific View, these Views are placed inside ViewHolders. Each ViewHolder can have up to one View and and the ViewHolder is used to display a particular View of an item within your RecyclerView.
For performance reasons, the RecyclerView will reuse ViewHolders for displaying Views by recycling ViewHolders that contain Views that shift offscreen with Views that shift onscreen when scrolling occurs.
The RecyclerView Adapter contains methods for creating and binding the ViewHolder called onCreateViewHolder(…) and onBindViewHolder(…) to enable this ViewHolder recycling to occur.
RecyclerView Adapter
ViewHolders are managed by RecyclerView adapters by creating the Views and ViewHolders and binding the ViewHolder to an item in the list.
Adapters notify the RecyclerView on specific changes to the data. Adapters can also handle interactions with Items such as clicks.
In addition to notifyDataSetChange(…) which is also supported by ListView for advising on changes to the date in the adapter, RecyclerView adapters also support the following methods:
- notifyItemInserted(…)
- notifyItemChanged(…)
- notifyItemRemoved(…)
These methods available within RecyclerView (not supported in ListView) should be utilised over notifyDataSetChanged(…) where possible to enable RecyclerView animations to work correctly.
ItemAnimator
Given the RecyclerView Adapter can notify when particular items in the dataset have been changed, added or removed this opens up the opportunity to implement more accurate animations of Items within the RecyclerView.
The ItemAnimator class simplifies the addition of animations to your RecyclerView. The ItemAnimator supports animating your RecyclerView on events such as:
- Appearance of list
- Disappearance of list
- Removal of Item
- Addition of Item
- Move of Item
- Change of Item
ItemDecoration
By default the RecyclerView will not create borders or dividers between items. If you want the add custom drawings to your RecyclerView canvas this can be supported using ItemDecoration.
Use the addItemDecoration(ItemDecoration) method on the RecyclerView to add an ItemDecoration. You can add multiple ItemDecorations to the same RecyclerView using the addItemDecoration(itemDecoration) method for each ItemDecoration.
You can create a margin around your item Views within a RecyclerView using the getItemsOffset(…) method
You can draw underneath the item Views within a RecyclerView using the onDraw(…) method
You can draw over the top of the item Views within a RecyclerView using the onDrawOver(…) method
RecycledViewPool
RecycledViewPool lets you share Views between multiple RecyclerViews. This comes in handy in scenarios where you have a nested RecyclerView and will provide a boost to your apps performance.
Use the setRecycledViewPool(RecycledViewPool) method on the multiple RecyclerViews providing the same instance of RecycledViewPool to share the pool.
RecyclerView automatically creates a RecycledViewPool if you don’t provide one.
ItemTouchHelper
ItemTouchHelper enables you to implement drag and drop and swipe to dismiss functionality against your RecyclerView.
What is a ListView?
ListView has been around since API version 1 and is used to display a list of items that can be scrolled vertically.
A ListView is an adapter view and uses a ListAdapter to create and populate the item Views.
ArrayAdapter and SimpleCursorAdapter are examples of adapters than can be used to provide the items to the ListView by using the setAdapter(ListAdapter) method and passing the Adapter to bind data the ListView.
ArrayAdapter can be used when you have a collection of Strings you would like displayed in a ListView.
SimpleCursorAdapter can be used when you have data coming from a Cursor such as results of a database query or content provider you want displayed in a ListView.
ListView does has some limited performance optimisation built into it. It will create and lay out the Views in the list as the user can see them on screen and then will lay out additional Views as the user scrolls. The adapter on the ListView will reuse Views of the same type where possible.
Conclusion
Summary
- RecyclerView has a mandatory use of the ViewHolder pattern providing better performance over ListView that requires customisation to support the ViewHolder pattern
- RecyclerView has greater support for LayoutManagement including vertical lists, horizontal lists, grids and staggered grids. ListView only supports vertical lists.
- ListView starts by default with dividers between items and requires customisation to add decorations. ItemDecoration class is available for RecyclerView to add drawings on top or underneath Items as well adding margins.
- RecyclerView adapters can inform you which items in your data has changed not just that your dataset has changed
- Due to the RecyclerView Adapters enabling us to be more specific about the data being changed, animations in RecyclerView are substantially easier to implement through the ItemAnimator compared to ListView
Conclusion
In the vast majority of cases where you need to show a list or grid of items that need to be scrolled vertically or horizontally you should I would recommend leveraging the more modern RecyclerView over ListView.
It is an absolute must have if you:
- You have a large data set you want to show in a list
- You have a non simplistic View of each Item in your list (e.g. has images, or multiple different sized TextViews etc.)
- You want accurate animations of the item and/or list
- You want custom decorations to be shown against with your item
The ListView may be still appropriate to use in cases where you have a small list of items to display in a vertical list in the user interface for example an overflow menu for Settings. Another example might be if you have legacy code using ListView where it is not worth upgrading to RecyclerView due to the small number of items being shown and the simplicity of the item’s appearance in the list.
Additional Sources
Some of the research on this article came from a great video published on the Android Developers YouTube channel at the 2016 Google I/O Conference, where Adam Powell and Yigit Boyar go into details of the functionality of RecyclerView, the history of ListView as well as the key differences and improvements pf RecyclerView from ListView.
ListView documentation on developer.android.com https://developer.android.com/reference/android/widget/ListView
RecyclerView documentation on developer.android.com https://developer.android.com/reference/android/support/v7/widget/RecyclerView
RecyclerView guide on developer.android.com https://developer.android.com/guide/topics/ui/layout/recyclerview