In the user interface of your Android app you can use gravity and layout_gravity attributes to align widgets in your layout but sometimes it can be difficult to remember which attribute to use.
The difference between the gravity and layout_gravity XML attributes in Android is that the android:gravity attribute is used to arrange the position of the content inside a View (for example text inside a Button widget) while the android:layout_gravity is used to arrange the position of the entire View relative to it’s container.
In this post I have documented the possible values for the layout_gravity and gravity attributes in Android. Also I have included code samples and screenshots showing how to use the layout_gravity and gravity XML attributes for the TextView, Button and EditText widgets.
I have also added section on how to set the gravity of views programmatically using the setGravity(…) method.
The code samples shared in this blog post are also available in a public GitHub repository I created at the link below.
https://github.com/learntodroid/AndroidGravityLayoutGravity
What are the possible values for the layout_gravity attribute in Android?
I have created the table below summarising the different attribute values available for layout_gravity in Android.
Attribute Value for “layout_gravity” | Description |
---|---|
left | Position the view to the left of it’s container |
right | Position the view to the right of it’s container |
start | Position the view to the beginning of it’s container |
end | Position the view to the end of it’s container |
top | Position the view to the top of it’s container |
bottom | Position the view to the bottom of it’s container |
center | Position the view to the center of it’s container in both the vertical and horizontal directions |
center_horizontal | Position the view to the center of it’s container in only the horizontal direction |
center_vertical | Position the view to the center of it’s container in only the vertical direction |
fill | Scale the view in the horizonal and vertical direction so that it fills it’s container |
fill_horizontal | Scale the view in only the horizontal direction so that it fills it’s container |
fill_vertical | Scale the view in only the vertical direction so that it fills it’s container |
clip_horizontal | Clip the left and/or right side(s) of the view within it’s container |
clip_vertical | Clip the top and/or bottom side(s) of the view within it’s container |
Multiple attribute values can be used together when setting the attribute value for android:layout_gravity by using the pipe “|” delimiter.
For example the following screenshot shows a TextView inside a LinearLayout with the android:layout_gravity attribute on the TextView set to “top|right”.
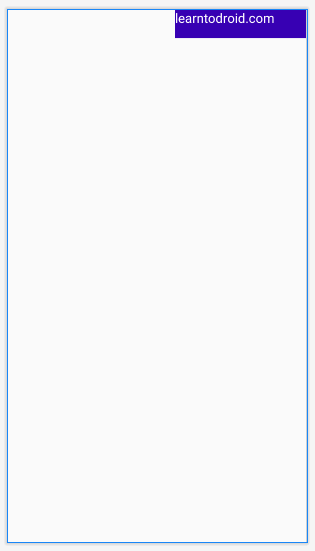
See the code sample for the layout resource file below.
What are the possible values for gravity attribute in Android?
I have created the table below summarising the different attribute values available for gravity in Android.
Attribute Value for “gravity” | Description |
---|---|
left | Position the content inside the view to the left |
right | Position the content inside the view to the right |
start | Position the content inside the view to the start |
end | Position the content inside the view to the end |
top | Position the content inside the view to the top |
bottom | Position the content inside the view to the bottom |
center | Position the content inside the view to the center in both the vertical and horizontal directions |
center_horizontal | Position the content inside the view to the center in only the horizontal direction |
center_vertical | Position the content inside the view to the center only the vertical direction |
fill | Scale the content inside the view in the horizonal and vertical direction so that it fills it’s container |
fill_horizontal | Scale the content inside the view in only the horizontal direction so that it fills it’s container |
fill_vertical | Scale the content inside the view in only the vertical direction so that it fills it’s container |
clip_horizontal | Clip the left and/or right side(s) of the content inside the view |
clip_vertical | Clip the top and/or button side(s) of the content inside the view |
Multiple attribute values can be used together when setting the attribute value for android:gravity by using the pipe “|” delimiter.
For example the following screenshot shows a TextView inside a LinearLayout with the android:gravity attribute on the TextView set to “bottom|right”.
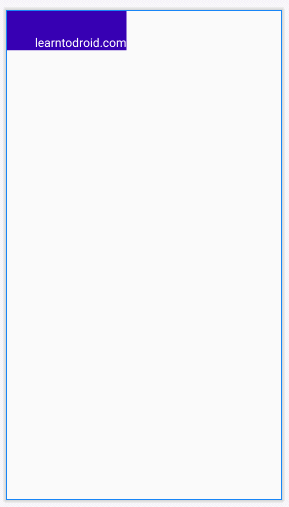
See the code sample for the layout resource file below.
How to use layout_gravity and gravity with a TextView in Android
When using a TextView widget in an Android app you can use the layout_gravity and gravity attributes to change the look and feel of the TextView.
The layout_gravity attribute can be used to position the TextView as a whole. The gravity attribute can be used to position the text inside the TextView.
See the screenshot below showing the use of the layout_gravity attribute and the gravity attribute for the TextView widget.
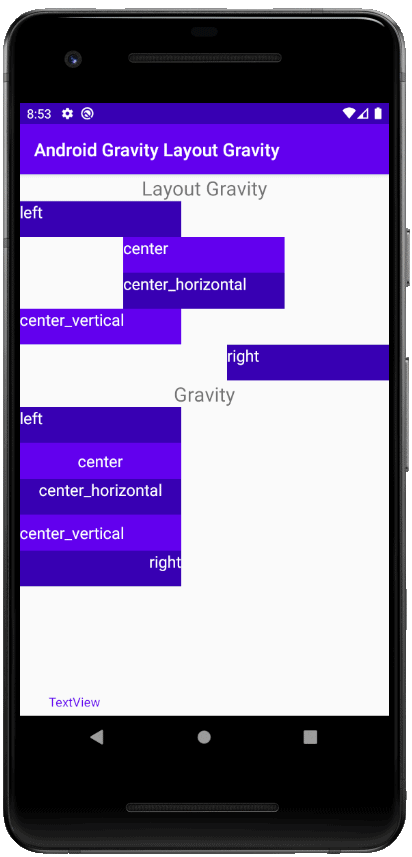
The screenshot above is built based on the layout resource below.
How to use layout_gravity and gravity with a Button in Android
When using a Button widget in an Android app you can use the layout_gravity and gravity attributes to change the look and feel of the Button.
The layout_gravity attribute can be used to position the Button as a whole with the Button text centered in side it. The gravity attribute can be used to position the text inside the Button.
See the screenshot below showing the use of the layout_gravity attribute and the gravity attribute for the Button widget.
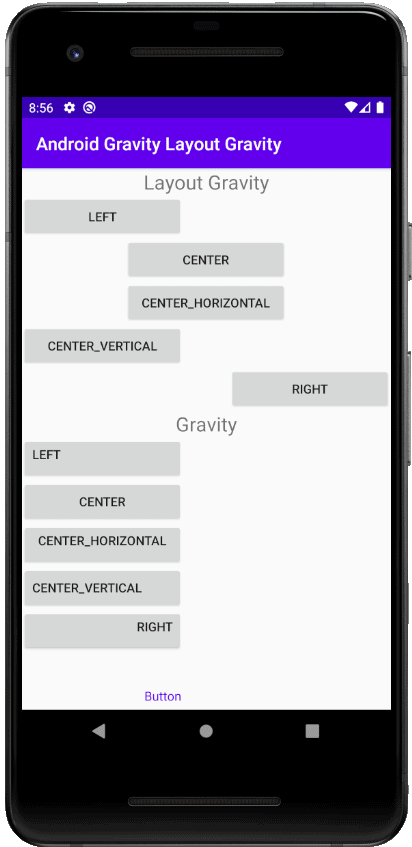
The screenshot above is built based on the layout resource below.
How to use layout_gravity and gravity with an EditText in Android
When using a EditText widget in an Android app you can use the layout_gravity and gravity attributes to change the look and feel of the EditText.
The layout_gravity attribute can be used to position the EditText as a whole. The gravity attribute can be used to position the EditText hint inside the EditText.
See the screenshot below showing the use of the layout_gravity attribute and the gravity attribute for the EditText widget.
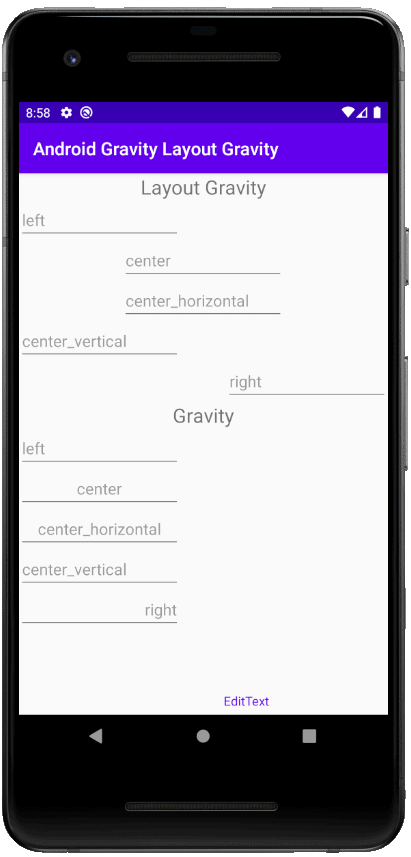
The screenshot above is built based on the layout resource below.
How to set gravity programmatically in Android
You can also set the gravity for a View programmatically in Android using the setGravity(…) method.
The setGravity(…) method accepts an integer as a parameter which can be one of the constants available on the following page in the official docs for gravity.
See the code sample below setting the gravity on a TextView using the setGravity(…) method.
The code sample above for setting the gravity on a TextView widget programmatically will produce the following screenshot.
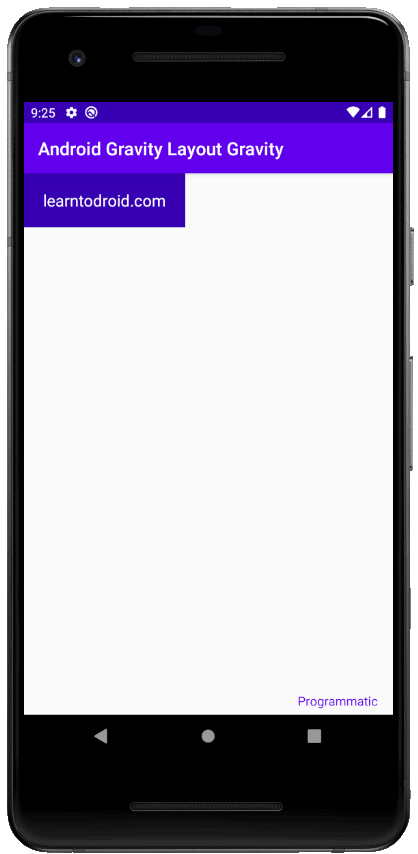